Offline remote data handling
Dealing with offline remote data is fundamental to ensuring data synchronization and consistency between the mobile app and the remote data source, allowing users to continue using the app and performing actions without interruption. Queue operations provide the functionality needed when the device regains network connectivity and manages a sequence of elements in a specific order. The commands in the queue can be manipulated to reduce the number of calls to the remote data store.
- Data Capture Offline:
- When the mobile app is offline, any actions that require remote data interaction, such as submitting a form, uploading a file, or syncing data, are queued.
- Each action is captured and stored in a queue in the local data provider on the device.
- Network Monitoring:
- The app monitors the network status. It triggers the dequeuing process when it detects that the device has regained connectivity.
- Dequeue and Sync:
- The app starts processing the commands in the queue. It dequeues each command and attempts to send it to the remote server.
- If the operation is successful, the app removes the command from the queue.
In the execute-entity, execute-entities , and submit-form actions, the queueOperation property is configured to determine how the record must be handled in the queue when the device is offline. There are two configuration options:
Property | description |
---|---|
replace | All queued commands for the specified record and method type are replaced with the current action. For example, a record is created and then updated a few times. Using replace for the update method results in only two commands in the queue for the record: create and update. If replace is not used, there will be a command for every action: create, update, update, update. Replace is recommended for backend systems with rate limits. The queueOperation: replace requires an id (must be in lowercase). This can either be configured in the:
|
add | All commands are added to the queue. When the queueOperation property is omitted, the default is add. |
Examples of configuring the required id property when using queueOperation: replace.
For scenarios where operations on a record must be treated as draft and all queued commands must be removed without impacting the local record, use the clear-queue action, and specify the id of the record and a title for the action. See clear all commands in the queue example.
When using the replace property with a delete method, all commands on the queue for the specified record are removed. The delete method will still delete the local entity record as expected, for example while offline a record is created with a tempId, then updated, and then deleted with a queueOperations: replace, the commands for that record are removed from the queue and local entity will also be deleted. This avoids the need for the full cycle of calls to be sent to the backend (create, update, delete) if the end result is that the record is deleted.
If the record to be delete has a valid Id then the queueOperations: add is used to add the record to the queue, when the device is back online the queue is processed and the record is deleted using the function.
If you want to cater for both tempId and avalid Id records when offline in one queueOperation configuration use the following expression =$isTempId(@ctx.current.item.id) ? replace:add
All tempIds for a record are replaced in all other queued commands if a valid id is returned. If you use a record's id in another record while offline and a valid id is returned back when the device is back online, updates the tempId used in all the other records that used it with the valid id. This makes for smoother integration with backend systems as the ids will match up. See working with REST ids for more information on returning the id.
In this example, when the device is offline and a customer record is created and then updated multiple times, only one create and one update command is queued. When the device is back online the queue is cleared. The remote data store returns an id that we can use to map back to the record locally in the outputTransform of the function (rest-create-customer). queueOperation is not required for the create of the customer because once the device comes online, the record will be created, and the id from the remote data store will be returned and any records with the same tempId will be updated with the returning id and will update the correct record. The queueOperation: replace is rather used in the update-customer jig.
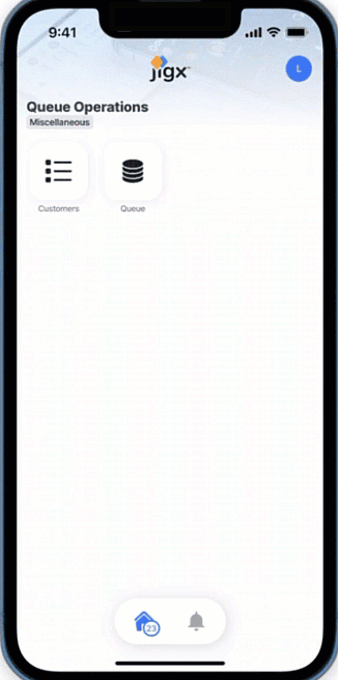
In this example, when the device is offline and a customer record is updated multiple times , all the update commands are queued. When the device is back online the queue is cleared.
In this example, when the device is offline and a customer record is updated multiple times and then deleted, all the the commands for the record are removed from the queue and local entity is deleted. When the device is back online the queue is cleared.
In this example, the remote data store does not return an id, and we need to sync the data before we get the correct backend id for the record. We need to be careful not to create and update the same record on the queue because the backend cannot associate the records after the sync. To accomodate for this in the update-customer we configure two execute-entity actions.
- The first action checks to see if a record has a tempId by using the following expression when: =$isTempId(@ctx.jig.inputs.customer.id). If the record on the queue has a tempId, we replace it using the create method with a new item that will be placed on the queue.
- The second action checks to see if the record has a valid Id rather than a tempId by using the following expression when: =$not($isTempId(@ctx.jig.inputs.customer.id)). If the record on the queue has a valid id, we replace it using the update method with an item that will be placed on the queue.
In this example, a secondary button is added to clear the queue for all commands using the action.clear-queue.
As you add the queueOperation property to actions, it is helpful to test or debug that the commands are being executed as configured. Here is a that can help you see the commands being queued when the device is offline, and then see the queue clear when the device is back online.
